Adaptive icons require API 26 or above. We use a combination of legacy icons as well as adaptive icons to serve users who are using Android versions below 26 as well as those using 26 or above. Android will use Adaptive icons for API 26 or above and fallback to use legacy icons for devices with API below 26.
For this tutorial we will create icon set using an online service https://easyappicon.com/.
Create icon set using the service and download the set. You should see the icons in two folders; Android and iOS.
For his tutorial we will use Android icon set. Go inside the folder and you would see folders as following
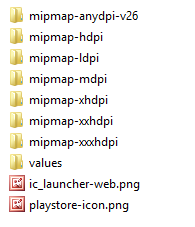
Let us also create an Android app using Cordova as described in the previous article
https://www.netexl.com/blog/use-cordova-to-compile-html5-games-to-android-app/
Create a new app by using the command as following
cordova create Sample com.mygames.sample Sample
A basic skeleton cordova project is created
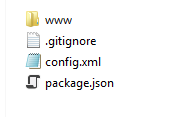
This is the bare min config.xml generated by cordova
<?xml version='1.0' encoding='utf-8'?>
<widget id="com.mygames.sample" version="1.0.0" xmlns="http://www.w3.org/ns/widgets" xmlns:cdv="http://cordova.apache.org/ns/1.0">
<name>Sample</name>
<description>
A sample Apache Cordova application that responds to the deviceready event.
</description>
<author email="dev@cordova.apache.org" href="http://cordova.io">
Apache Cordova Team
</author>
<content src="index.html" />
<access origin="*" />
<allow-intent href="http://*/*" />
<allow-intent href="https://*/*" />
<allow-intent href="tel:*" />
<allow-intent href="sms:*" />
<allow-intent href="mailto:*" />
<allow-intent href="geo:*" />
<platform name="android">
<allow-intent href="market:*" />
</platform>
<platform name="ios">
<allow-intent href="itms:*" />
<allow-intent href="itms-apps:*" />
</platform>
</widget>
Add some preferences for the Android platform in config.xml
<platform name="android">
<allow-intent href="market:*" />
<preference name="android-minSdkVersion" value="23"/>
<preference name="android-targetSdkVersion" value="29"/>
<preference name="android-installLocation" value="auto"/>
<preference name="android-signed" value="true"/>
<preference name="Orientation" value="portrait"/>
<preference name="AndroidLaunchMode" value="singleTop"/>
</platform>
Now we are going to create a new folder in our cordova project “package-assets”.
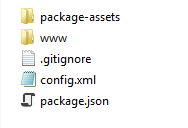
Copy the entire iconset folder created earlier in the package-assets folder and add configuration entries for these in config.xml as following
<resource-file src="package-assets/values/ic_launcher_background.xml" target="app/src/main/res/values/ic_launcher_background.xml" />
<icon background="@color/ic_launcher_background" density="ldpi" foreground="package-assets/mipmap-ldpi/ic_launcher.png" />
<icon background="@color/ic_launcher_background" density="mdpi" foreground="package-assets/mipmap-mdpi/ic_launcher_foreground.png" src="package-assets/mipmap-mdpi/ic_launcher.png" />
<icon background="@color/ic_launcher_background" density="hdpi" foreground="package-assets/mipmap-hdpi/ic_launcher_foreground.png" src="package-assets/mipmap-hdpi/ic_launcher.png"/>
<icon background="@color/ic_launcher_background" density="xhdpi" foreground="package-assets/mipmap-xhdpi/ic_launcher_foreground.png" src="package-assets/mipmap-xhdpi/ic_launcher.png"/>
<icon background="@color/ic_launcher_background" density="xxhdpi" foreground="package-assets/mipmap-xxhdpi/ic_launcher_foreground.png" src="package-assets/mipmap-xxhdpi/ic_launcher.png"/>
<icon background="@color/ic_launcher_background" density="xxxhdpi" foreground="package-assets/mipmap-xxxhdpi/ic_launcher_foreground.png" src="package-assets/mipmap-xxxhdpi/ic_launcher.png"/>
I have used a background color here but if you want to use a background image that can also be done. Refer to the cordova reference link below for more details. Adaptive icons are a combination of background and foreground images or, background color and foreground image as per your configuration for API 26 or more. If you specify src attribute, it will be used for fallback icon on API less than 26. If src attribute is not mentioned, then foreground image is used for the icon on devices with API less than 26. The src
attribute is not used for adaptive icons
After all updates our config.xml looks like the following now
<?xml version='1.0' encoding='utf-8'?>
<widget id="com.mygames.sample" version="1.0.0" xmlns="http://www.w3.org/ns/widgets" xmlns:cdv="http://cordova.apache.org/ns/1.0">
<name>Sample</name>
<description>
A sample Apache Cordova application that responds to the deviceready event.
</description>
<author email="dev@cordova.apache.org" href="http://cordova.io">
Apache Cordova Team
</author>
<content src="index.html" />
<access origin="*" />
<allow-intent href="http://*/*" />
<allow-intent href="https://*/*" />
<allow-intent href="tel:*" />
<allow-intent href="sms:*" />
<allow-intent href="mailto:*" />
<allow-intent href="geo:*" />
<platform name="android">
<allow-intent href="market:*" />
<preference name="android-minSdkVersion" value="23"/>
<preference name="android-targetSdkVersion" value="29"/>
<preference name="android-installLocation" value="auto"/>
<preference name="android-signed" value="true"/>
<preference name="Orientation" value="portrait"/>
<preference name="AndroidLaunchMode" value="singleTop"/>
<resource-file src="package-assets/values/ic_launcher_background.xml" target="app/src/main/res/values/ic_launcher_background.xml" />
<icon background="@color/ic_launcher_background" density="ldpi" foreground="package-assets/mipmap-ldpi/ic_launcher.png" />
<icon background="@color/ic_launcher_background" density="mdpi" foreground="package-assets/mipmap-mdpi/ic_launcher_foreground.png" src="package-assets/mipmap-mdpi/ic_launcher.png" />
<icon background="@color/ic_launcher_background" density="hdpi" foreground="package-assets/mipmap-hdpi/ic_launcher_foreground.png" src="package-assets/mipmap-hdpi/ic_launcher.png"/>
<icon background="@color/ic_launcher_background" density="xhdpi" foreground="package-assets/mipmap-xhdpi/ic_launcher_foreground.png" src="package-assets/mipmap-xhdpi/ic_launcher.png"/>
<icon background="@color/ic_launcher_background" density="xxhdpi" foreground="package-assets/mipmap-xxhdpi/ic_launcher_foreground.png" src="package-assets/mipmap-xxhdpi/ic_launcher.png"/>
<icon background="@color/ic_launcher_background" density="xxxhdpi" foreground="package-assets/mipmap-xxxhdpi/ic_launcher_foreground.png" src="package-assets/mipmap-xxxhdpi/ic_launcher.png"/>
</platform>
<platform name="ios">
<allow-intent href="itms:*" />
<allow-intent href="itms-apps:*" />
</platform>
</widget>
Now let us simply run following commands to build an APK and test it
cd Sample
cordova platform add android
cordova build android
Ref Links: https://cordova.apache.org/docs/en/dev/config_ref/images.html#android